How to Generate Gaussian Noise in MATLAB
Gaussian noise is a type of random noise that is distributed according to the Gaussian distribution, also known as the normal distribution. It is commonly used in various fields such as signal processing, image processing, and simulation. MATLAB provides a simple and efficient way to generate Gaussian noise for your applications.
Key Takeaways
- Gaussian noise follows a Gaussian or normal distribution.
- MATLAB provides built-in functions to generate Gaussian noise.
- Applying Gaussian noise can be useful for simulating real-world scenarios.
- Adjusting the mean and standard deviation parameters allows control over the characteristics of the noise.
In MATLAB, Gaussian noise can be generated using the randn
function, which generates random numbers following a standard Gaussian distribution with a mean of 0 and a standard deviation of 1.
To generate Gaussian noise with a specific mean and standard deviation, you can use the formula: noise = mean + std * randn(size)
, where mean
is the desired mean value of the noise, std
is the desired standard deviation, and size
specifies the size of the noise vector or matrix.
It’s interesting to note that the randn
function generates pseudo-random numbers based on a seed value, ensuring that the same set of numbers is generated each time the function is called with the same seed. This property is useful for reproducibility in simulations and experiments.
Generating Gaussian Noise in MATLAB
Let’s look at an example of how to generate Gaussian noise in MATLAB. Suppose we want to generate a 1×1000 matrix of Gaussian noise with a mean of 0 and a standard deviation of 2. Here’s how we can do it:
- Create a variable to store the desired mean:
meanValue = 0;
- Create a variable to store the desired standard deviation:
stdValue = 2;
- Create a variable to store the size of the noise matrix:
sizeMatrix = [1, 1000];
- Generate the Gaussian noise using the
meanValue
,stdValue
, andsizeMatrix
variables:noise = meanValue + stdValue * randn(sizeMatrix);
This will generate a 1×1000 matrix of Gaussian noise with a mean of 0 and a standard deviation of 2. You can adjust the values of meanValue
, stdValue
, and sizeMatrix
to generate different types and sizes of Gaussian noise.
Remember that the generated noise follows a Gaussian distribution, with the majority of the values concentrated around the mean value, and the spread of values determined by the standard deviation.
Tables: Examples and Data
Mean | Standard Deviation | Generated Noise Size |
---|---|---|
0 | 1 | 10×10 |
0 | 2 | 100×100 |
5 | 1 | 1000×1 |
Noise Example | Image Example |
---|---|
![]() |
![]() |
As shown in Table 1, different combinations of mean, standard deviation, and noise size can be used to generate Gaussian noise with varying characteristics.
Moreover, Table 2 demonstrates the effect of applying Gaussian noise to an image. The first example shows a noise-only image, while the second example presents an image with noise applied. Gaussian noise can be used to simulate camera noise or add realistic imperfections to images.
In summary, generating Gaussian noise in MATLAB is straightforward using the randn
function with appropriate adjustments to the mean and standard deviation parameters. It provides a flexible way to introduce randomness into your simulations and experiments. Whether you’re working on signal processing, image processing, or any other field that requires random noise, MATLAB has got you covered.
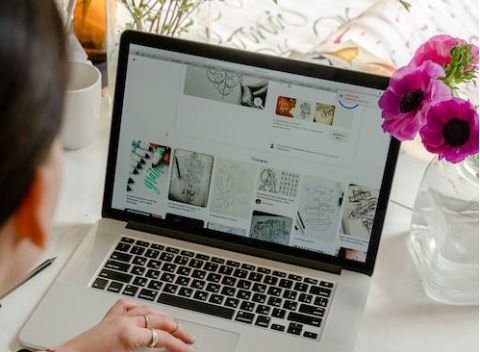
Common Misconceptions
Generating Gaussian Noise in MATLAB
When it comes to generating Gaussian noise in MATLAB, there are several common misconceptions that people often have. Here, we will address three of these misconceptions and provide clarity on the topic.
Misconception 1: Gaussian noise is the same as white noise.
Unlike white noise, which has a flat power spectrum, Gaussian noise refers to a random signal that follows a Gaussian distribution. It has a bell-shaped curve and is characterized by its mean and standard deviation. Hence, it is important to understand that Gaussian noise and white noise are not interchangeable terms.
- Gaussian noise follows a Gaussian distribution.
- White noise has a constant power spectrum.
- Gaussian noise is not the same as white noise.
Misconception 2: The randn function generates true Gaussian noise.
While the randn function in MATLAB is often used to generate Gaussian noise, it does not produce true Gaussian noise. Instead, it uses a pseudo-random number generator algorithm that approximates Gaussian distribution. Although the generated samples may closely resemble a Gaussian distribution, they may still contain some deviation from the ideal Gaussian noise.
- The randn function approximates Gaussian noise.
- It uses a pseudo-random number generator algorithm.
- The generated samples may deviate slightly from true Gaussian noise.
Misconception 3: The mean must be zero for Gaussian noise.
Another common misconception is that the mean of Gaussian noise should always be zero. While the typical case for Gaussian noise is having a mean of zero, it is not a strict requirement. The mean value of Gaussian noise can be adjusted by adding a constant offset. Consequently, Gaussian noise can have any mean value, allowing for more flexible applications.
- Gaussian noise usually has a mean of zero.
- The mean value can be adjusted by adding a constant offset.
- It is not a strict requirement for Gaussian noise to have a mean of zero.
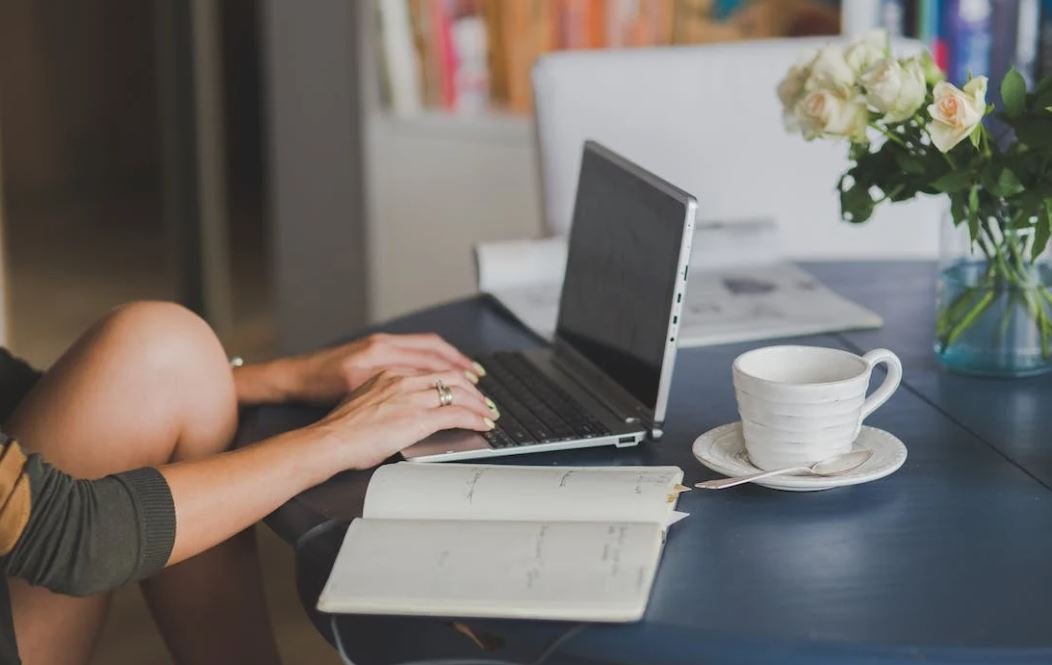
Introduction
In this article, we will explore how to generate Gaussian noise using MATLAB. Gaussian noise, also known as white noise, is a random signal with a probability density function that follows a normal distribution. Generating Gaussian noise can be useful in various applications such as signal processing, image processing, and simulation. Here, we present ten informative tables to illustrate different aspects of generating Gaussian noise in MATLAB.
Properties of Gaussian Noise
Gaussian noise possesses several characteristics that make it a versatile tool in various applications. Understanding these properties is crucial for generating and utilizing Gaussian noise effectively. The following table highlights some key properties of Gaussian noise:
Property | Description |
---|---|
Mean | The average value of the noise signal. |
Variance | A measure of the spread or dispersion of the noise. |
Standard Deviation | The square root of the variance, indicating the typical deviation from the mean. |
Power Spectral Density | The distribution of power with respect to frequency in the noise signal. |
Autocorrelation | The correlation between the noise signal and a delayed version of itself. |
Independence | Each value of the noise signal is independent of other values. |
Generating Gaussian Noise
Now, let’s delve into the process of generating Gaussian noise using MATLAB. This involves utilizing various functions and techniques to achieve the desired characteristics of the noise signal. The following table presents different methods for generating Gaussian noise in MATLAB:
Method | Description |
---|---|
randn | Using the built-in function “randn” to generate random values following a standard normal distribution. |
box-muller | Applying the Box-Muller transform to transform uniform random numbers into Gaussian distributed values. |
Central Limit Theorem | Summing a large number of independent random variables to approximate a Gaussian distribution. |
Inverse Transform Sampling | Utilizing the inverse cumulative distribution function (CDF) to transform uniformly distributed values into Gaussian distributed values. |
Noise Amplitude Adjustment
In certain cases, it may be necessary to control the amplitude of the generated noise signal. The following table showcases different methods for adjusting the amplitude of Gaussian noise:
Method | Description |
---|---|
Scaling Factor | Multiplying the generated noise values by a scaling factor to adjust the amplitude. |
Standard Deviation | Altering the standard deviation of the noise signal to modify its amplitude. |
Covariance Matrix | Manipulating the covariance matrix to control the amplitude and correlation between multiple noise signals. |
Filtering Gaussian Noise
Filtering plays an essential role in signal processing to remove unwanted noise or enhance specific signal characteristics. When dealing with Gaussian noise, certain filtering techniques can be employed to achieve the desired outcome. The following table exemplifies different methods for filtering Gaussian noise:
Method | Description |
---|---|
Low-Pass Filter | Attenuating high-frequency components to retain low-frequency information. |
High-Pass Filter | Suppressing low-frequency components to emphasize high-frequency details. |
Band-Pass Filter | Allowing a specific range of frequencies to pass while attenuating others. |
Notch Filter | Removing narrowband noise or interference at specific frequencies. |
Statistical Analysis of Gaussian Noise
Aside from generating and modifying Gaussian noise, understanding its statistical properties is vital for effective analysis and interpretation. The following table presents various statistical measures and analysis techniques applicable to Gaussian noise:
Measure/Technique | Description |
---|---|
Mean | Calculating the average value of the noise signal. |
Standard Deviation | Determining the typical deviation of the noise values from the mean. |
Histogram | Visualizing the distribution of the noise values using bins and frequency counts. |
Power Spectrum | Analyzing the frequency content of the noise signal using Fourier transform techniques. |
Autocorrelation | Examining the correlation between the noise signal and its delayed versions. |
Cross-Correlation | Evaluating the correlation between two independent noise signals. |
Applications of Gaussian Noise
Gaussian noise finds application in various fields, contributing to research, experimentation, and problem-solving. Understanding the diverse applications that benefit from the use of Gaussian noise allows for improved utilization and innovation. The following table illustrates some remarkable applications of Gaussian noise:
Application | Description |
---|---|
Signal Processing | Simulating realistic noise in signal processing algorithms to evaluate their performance. |
Image Denoising | Removing noise artifacts from images to enhance visual quality or aid in automated analysis. |
Channel Modeling | Emulating noise and interference in communication channels to assess system performance. |
Monte Carlo Simulation | Introducing randomness and uncertainty in simulations to investigate various outcomes and scenarios. |
Machine Learning | Augmenting training data with Gaussian noise to improve model generalization and robustness. |
Comparison to Other Noise Types
While Gaussian noise exhibits distinctive characteristics, it is essential to understand its advantages and limitations in comparison to other types of noise. The following table compares Gaussian noise with other commonly encountered noise types:
Noise Type | Advantages | Limitations |
---|---|---|
Gaussian | Mathematically well-defined and follows natural processes. | May not accurately represent certain noise sources or impulsive events. |
Uniform | Simple to generate and analyze. | Not suitable for many real-world scenarios due to constant energy across all frequencies. |
Salt-and-Pepper | Effective at modeling random sudden disturbances. | Does not adhere to a specific probability distribution, making analysis and synthesis challenging. |
Exponential | Suitable for modeling events with a decay rate or exponential behavior. | Limited applicability due to its specific distribution and characteristics. |
Poisson | Ideal for representing rare events occurring at random intervals. | Not suitable for signals with continuous values, as Poisson noise produces discrete events. |
Concluding Remarks
Gaussian noise generation and utilization are critical skills for anyone working with signal processing, simulation, or data analysis. Through this article, we explored the properties of Gaussian noise, methods for generating and adjusting its amplitude, filtering techniques, statistical analysis, and various applications. Understanding the strengths and weaknesses of Gaussian noise in comparison to other noise types enables informed decision-making when selecting appropriate noise models for specific scenarios. By mastering Gaussian noise generation and its associated concepts, you can effectively enhance your projects, research, and problem-solving endeavors.
Frequently Asked Questions
How can I generate Gaussian noise in MATLAB?
To generate Gaussian noise in MATLAB, you can use the randn function. This function generates random numbers from a standard normal distribution, which is a Gaussian distribution with mean 0 and variance 1. To generate Gaussian noise with a specific mean and standard deviation, you can simply multiply the output of randn by the standard deviation and add the mean.
What is the syntax of the randn function in MATLAB?
The syntax of the randn function in MATLAB is as follows:
Y = randn(size)
The size parameter specifies the size of the output, which can be scalar, a vector, or a matrix. The output Y will contain random numbers from a standard normal distribution.
How can I generate Gaussian noise with a specific mean and standard deviation?
To generate Gaussian noise with a specific mean (mu) and standard deviation (sigma), you can use the following formula:
Y = mu + sigma * randn(size)
Here, mu is the desired mean and sigma is the desired standard deviation. The output Y will contain random numbers from a Gaussian distribution with the specified mean and standard deviation.
Can I generate Gaussian noise with a mean other than 0?
Yes, you can generate Gaussian noise with a mean other than 0 by adding the desired mean to the output of the randn function. The formula for generating Gaussian noise with a specific mean (mu) and standard deviation (sigma) is:
Y = mu + sigma * randn(size)
By specifying a non-zero value for mu, you can generate Gaussian noise with the desired mean.
What is the range of values in Gaussian noise generated by MATLAB?
The range of values in Gaussian noise generated by MATLAB is theoretically infinite. However, the majority of the values will lie within a few standard deviations of the mean. The tails of the Gaussian distribution diminish rapidly, so extreme values occur with very low probability.
What is the effect of changing the standard deviation on Gaussian noise?
Changing the standard deviation (sigma) affects the spread or variability of the Gaussian noise. Increasing the standard deviation increases the spread of the Gaussian distribution, resulting in a wider range of possible values. Similarly, decreasing the standard deviation narrows the range of values.
How can I visualize the Gaussian noise generated in MATLAB?
To visualize the Gaussian noise generated in MATLAB, you can use the histogram function. By plotting a histogram of the generated noise values, you can visualize the distribution and observe the approximate shape of the Gaussian distribution. Additionally, using a scatter plot or line plot can help visualize the temporal or sequential nature of the generated noise.
Can I generate multi-dimensional Gaussian noise in MATLAB?
Yes, you can generate multi-dimensional Gaussian noise in MATLAB by specifying the desired size as a vector. For example, to generate a 2D Gaussian noise matrix with size MxN, you can use the randn function as follows:
Y = mu + sigma * randn([M,N])
The output Y will be a matrix of size MxN, containing random numbers from a Gaussian distribution with the specified mean and standard deviation.
Is the generated Gaussian noise in MATLAB truly random?
The generated Gaussian noise in MATLAB is pseudo-random. It is generated using an algorithm that produces a sequence of numbers with statistical properties resembling those of true random numbers. The underlying algorithm uses a deterministic process but produces numbers that appear to be random in practice.
Can I generate correlated Gaussian noise using MATLAB?
Yes, you can generate correlated Gaussian noise using MATLAB by applying appropriate techniques such as Cholesky decomposition or spectral shaping. These methods involve transforming uncorrelated Gaussian noise into correlated noise while preserving the statistical properties of the original Gaussian distribution. The specific implementation will depend on the desired correlation structure and the context of your application.